How To Login to Windows with your Phone's NFC
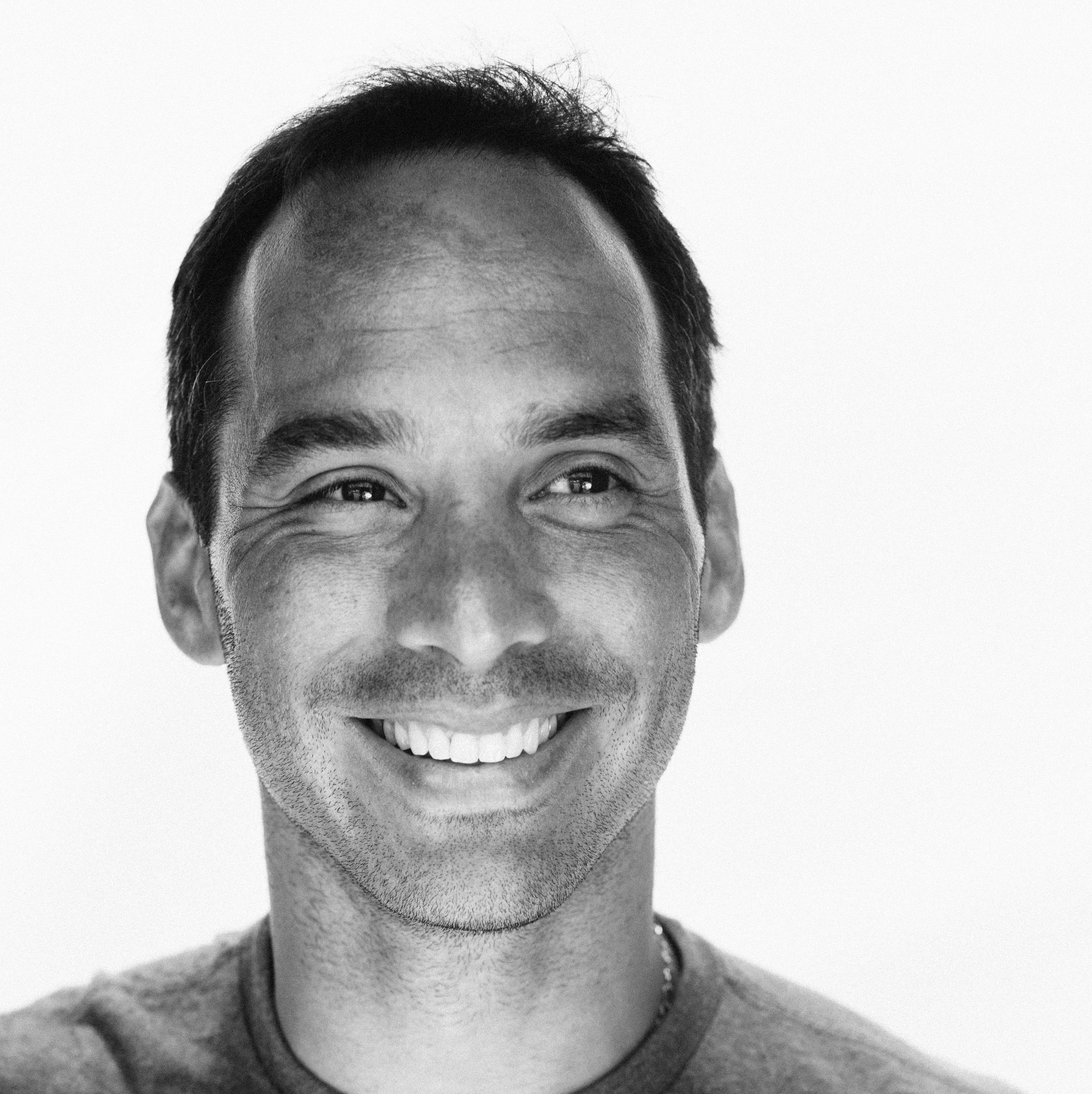
Updated December 17, 2024 06:19
Overview
There's times when you're forced to enter a long password so often that it's super inconvenient. In a recent poll of IT professionals, respondents cited "improved user convenience" as the top driver for system upgrades over "improved security posture" by an 11% margin. This tutorial walks through setting up a wallet pass and VTAP reader to securely login to a Windows computer with your phone's NFC chip. It'll involve running a background process that monitors the Lock screen and momentarily puts the VTAP in keyboard mode to summon the login prompt and inject the password when you scan your mobile wallet.
This tutorial assumes you've met the following pre-requisites:
- Windows computer or remote server
- Apple or Google Wallet pass with your Windows credentials issued by PassNinja
- VTAP series NFC reader properly configured for PassNinja passes
Manage Credentials Easy with PassNinja
Getting your phone's NFC to log you in to a Windows lock screen requires you to first put your login credentials into your mobile wallet. Whether for Apple or Google Wallet, PassNinja is a great tool to help you do this. Go to the PassNinja dashboard and create a new pass template for your mobile wallet with Access Control set as the style. You can design out the look of the pass but what's most important is to set the NFC message field with your Windows password and send yourself a sample pass to install it. If you get stuck, you can check this other guide on scanning static content in passes for more details.
To keep things simple, we put the Windows password in clear text in the NFC message field and without any other data. This is safe because calls to our API are point-to-point encrypted (TLS 1.3), our compute environment meets latest security requirements (SSAE-16 SOC2), and the Apple and Google Wallets encrypt (RSA:2048) and store NFC data in a protected area of your phone. The diagram below shows the data workflow and the several security protections in place. The red key exemplifies the NFC message data is as it's handled throughout the process.
Once you've issued and installed a pass with your Windows credentials, you're all set to move to the next step. Yet if you need to update your wallet pass with a new password or something, it's only a few API commands to do so. You'll need a few things from the PassNinja dashboard to do so, including your account id, your API key, your pass template id, and your issued pass id. Here's a couple screen shots of where to find each of these (highlighted in red) on your PassNinja dahboard:
You can use these variables to setup a script to send pass updates in your favorite programming language. We have SDKs for Javascript, Python, Ruby, PHP, C#, Java and Swift and the updates happen seamlessly and without any need to reinstall the pass. Here's an example of the script I use in Python:
import passninja
passninja_client = passninja.PassNinjaClient('**your-account-id**', '**your-api-key**')
simple_pass_object = passninja_client.passes.put(
'ptk_????', # pass template id (listed on dashboard->Get Started)
'pid_????', # pass id (listed on dashbaord->Logs)
{
"member-name": "Richard Grundy",
"nfc-message": "NewWindowsP@55w0rd",
} # pass data
) # installed passes will update automatically
That's it! Now you can easily manage your Windows Login pass and keep it in sync with your Windows credentials. Next we'll look at building an app to control a VTAP series NFC reader and enter your credentials into the Windows lock screen.
Coding a LoginBot app
There are a few things that need to happen to capture your credentials from your phone's NFC and get them to unlock Windows, starting with figuring out the right keystrokes to go through. The VTAP's keyboard mode can only emulate simple keystrokes (no key combos) represented by the ASCII character code chart (no function keys). So we'll need to study the Windows lock screen to see what keystrokes get us to the login prompt.
The Windows lock screen is managed by the LogonUI.exe process. When it's engaged, it appears to have 3 states: Screen off, Screen on with Time/News/Weather, and Screen on with password prompt selected. Hitting the Escape key (ESC) can cycle through these screens and always end at the password-prompt-selected state without generating any error conditions. To keep things simple, we'll assume the current user matches the wallet pass user but a similar approach can be used to login as a different user as well.
Since we don't want any keystrokes to be injected unless we're in the LogonUI.exe context, we'll need to monitor when the lock screen is launched and enable the VTAP's keyboard mode only during that time. We covered how to control the VTAP from a background app in another tutorial so we'll jump right into building our LoginBot app next. The gist of what LoginBot will do is:
- Open a COM port connection to the VTAP
- Initialize VTAP configurations with:
- Passive mode enabled to prevent log file from capturing passwords by mistake
- Keyboard Mode prefix set to ESC, ESC, ESC before injecting NFC message data
- Keyboard Mode delay set to 30ms to give LogonUI.exe time to respond to keystrokes
- Log responses to VTAP configurations
- Check for Windows lock screen state
- If lock screen is active,
- > enable VTAP Keyboard mode and log it Active
- Else lock screen is inactive,
- > disable VTAP Keyboard mode and log it Inactive
- Go to Step 4
Pretty straightforward, right? We'll use Windows PowerShell to code this up into the script shown below. You can copy/paste it into a text file and update it with your COM port identifier and log file path. Then save it as LoginBot.ps1 to run it from a PowerShell terminal.
$port = New-Object System.IO.Ports.SerialPort "COM5",115200
$port.DtrEnable = 1 # enable Data To Recieve
# initialize the VTAP with PassiveInterfaces=1, KBPrefix=%1B%1B%1B, KBDelayMS=30
$port.Open()
$port.Write("`$PassiveInterfaces=1`r`n") # Enable Passive mode on COM port
$port.Write("`$KBPrefix=%1B%1B%1B`r`n") # Add ESC, ESC, ESC before data
$port.Write("`$KBDelayMS=30`r`n") # Set keystroke latency to 30ms
Start-Sleep 2
$response = $port.ReadExisting() # log responses
$response = $response.Replace("`r`n", ' ')
Add-Content -Path "C:\Users\Richard Grundy\Documents\LoginLog.txt" -Value "$(Get-Date) : PassiveInterfaces, KBPrefix, KBDelayMS $response"
$port.Close()
while ($true) { # check for Lock screen
$process = @(Get-WmiObject -Class Win32_Process -Filter "Name = 'LogonUI.exe'" -ErrorAction SilentlyContinue | Where-Object {$_.SessionID -eq $([System.Diagnostics.Process]::GetCurrentProcess().SessionId)})
if ($process.Count -gt 0) { # if locked
$port.Open() # open COM port
$port.Write("`$KBLogMode=1`r`n") # enable KBLogMode
Start-Sleep 1
$response = $port.ReadExisting() # log response
$response = $response.Replace("`r`n", '')
Add-Content -Path "C:\Users\Richard Grundy\Documents\LoginLog.txt" -Value "$(Get-Date) : Active $response"
$port.Close() # close COM port
}
else { # if not locked
$port.Open() # open COM port
$port.Write("`$KBLogMode=0`r`n") # disable KBLogMode
Start-Sleep 1
$response = $port.ReadExisting() # log response
$response = $response.Replace("`r`n", '')
Add-Content -Path "C:\Users\Richard Grundy\Documents\LoginLog.txt" -Value "$(Get-Date) : Inactive $response"
$port.Close() # close COM port
}
}
Once you get it running, you can make it into a standalone application with this command:
Invoke-PS2EXE .\LoginBot.ps1 .\LoginBot.exe
This will let you double-click it to run it in the boackground or set it up to run automatically at startup in Windows.
Putting it all Together
Now that we have all the pieces, let's put it all together! Follow these steps to test out the experience:
- Windows machine logged in with Current User matching Window Login pass and VTAP connected over USB
- Launch LoginBot.exe and check that LoginLog.txt is being populated with Inactive OK
- Press CTRL-ALT-DEL and select Lock screen from menu
- Double-tap iPhone power button to launch Apple Wallet and authenticate with FaceID/TouchID/Passkey.
- Present iPhone top end to VTAP scan surface and what the magic happen!
- Once logged in, open any text editor and scan your pass again on the VTAP... you'll see nothing!
As passwords change (and get longer) you're just a simple API call away from updating your Windows Login pass. You can also modify LoginBot to monitor different applications that require different credentials and reconfigure the VTAP to scan for the right one when it's in context. It's more convenient and secure than most password manager services. 💡
Conclusion
PassNinja, LoginBot and a VTAP are a great solution for secure logon to systems where keyboard entry is the only option. The added convenience of an NFC tap and improved security of proof-of-presence biometrics (FaceID, TouchID, etc) makes for an efficient method of unlocking your workstation. Or any app for that mater. The programmability of a complete credential management system is made easy with the PassNinja API and helper libraries.
If you have any feedback on this article, let us know! Email content@passninja.com